Key Takeaways
- Accessibility essentials: Accessible HealthTech requires semantic HTML, screen reader compatibility, adequate touch targets, proper contrast ratios, keyboard navigation, and support for text resizing.
- Practical implementation: The article provides real code examples for proper HTML structure, ARIA attributes, focus management, color contrast solutions, and responsive text handling.
- WCAG compliance: Implementing the four core WCAG principles (Perceivable, Operable, Understandable, Robust) ensures applications work for users with diverse abilities and meet regulatory requirements.
- Testing approach: Effective accessibility requires both automated testing with tools like axe and manual testing with screen readers, keyboard navigation, and responsive design verification.
- Component-based strategy: Building accessibility into reusable components, focus management utilities, and adaptive interfaces from the beginning is more effective than retrofitting accessibility later.
Is Your HealthTech Product Built for Success in Digital Health?
.avif)
In healthcare, accessibility isn't a nice-to-have—it’s a must. But for developers, that often raises a tough question: What does accessibility actually look like in code? What do we need to do, exactly, to make sure our apps work for people with disabilities—and meet compliance requirements while we're at it?
This article is built around practical insights from one of our developers at Momentum. It's not theory. It's not marketing fluff. It's a clear, actionable breakdown of the exact steps and code-level details that go into building accessible HealthTech products.
Whether you're optimizing for screen readers, improving touch target sizing, or baking accessibility into your component library—this is the technical checklist you’ll want on hand.
.png)
TL;DR: Quick Checklist Summary
If you’re short on time, here’s what you need to hit:
- Use semantic HTML
- Test with screen readers
- Keep touch targets large
- Meet WCAG contrast ratios
- Ensure keyboard navigation
- Support text resizing
These are the high-level goals. The rest of this article breaks down how to achieve them.
Essential Front-End Development Considerations
Accessibility starts at the front-end layer. Many of the biggest blockers for users—especially those using assistive tech—are rooted in HTML structure, visual design, or input behavior. Let’s dig into each one.
Screen Reader Compatibility
Screen readers rely on clean, semantic markup to parse content. If you skip that step, everything else falls apart.
Semantic HTML Structure
Use proper HTML elements for their intended purposes, as we implemented in our healthcare application projects:
<!-- Instead of this -->
<div class="button" onclick="submitForm()">Submit</div>
<!-- Use this -->
<button type="submit">Submit</button>
Meaningful Alt Text
Provide descriptive alternative text for all images, describing images with alt text that captures what users need to know:
<img src="pill.jpg" alt="Red oval pill with score line, 10mg Lisinopril">
ARIA Attributes
Use ARIS only when standard, native HTML elements aren't sufficient:
<div role="button" aria-pressed="false" tabindex="0"
aria-label="Mark medication as taken">Mark as taken</div>
Focus Management
Make sure users know where they are and what they're interacting with—at all times. Tab order matters. Focus visibility matters.
Touch Targets and Motor Control
Think about your most dexterity-challenged user. Now ask: can they use this comfortably?
Many developers overlook this—especially if they’re designing for desktop-first. In HealthTech, that's a big mistake. Large touch targets (at least 44×44 pixels, but preferably larger for healthcare applications) and forgiving spacing reduce frustration and boost accuracy for everyone, but especially for older or motor-impaired users.
Visual Accessibility: Color and Contrast
Good contrast isn’t about branding. It’s about legibility in real-world lighting and vision conditions.
Make sure:
- You maintain a minimum contrast ratio of 4.5:1 for normal text (WCAG AA standard)
- Color isn’t the only signal (use icons, text, labels to convey information)
- You test using color blindness simulators
- You provide high-contrast mode options
.alert-message {
color: #d42020; /* Ensure this passes contrast checks */
border: 2px solid #d42020;
}
/* High contrast mode */
@media (prefers-contrast: high) {
.alert-message {
color: #000000;
border: 3px solid #000000;
}
}
Responsive Text and Layouts
Users will change their system font size. Your UI needs to adapt, not break.
This means:
- Use
rem
or%
instead ofpx
- Support system font size adjustments without breaking layouts
- Avoid fixed-sized containers that clip text when enlarged
- Consider offering in-app text size adjustments
body {
font-size: 100%; /* Base font size */
}
.medication-name {
font-size: 1.25rem; /* REM scales with user preferences */
line-height: 1.5;
}
.medication-card {
min-height: fit-content;
height: auto;
overflow: visible; /* Prevents clipping */
}
For in-app text size adjustment:
function adjustTextSize(sizePreference) {
const root = document.documentElement;
switch(sizePreference) {
case 'small': root.style.fontSize = '90%'; break;
case 'medium': root.style.fontSize = '100%'; break;
case 'large': root.style.fontSize = '120%'; break;
case 'extra-large': root.style.fontSize = '150%'; break;
}
localStorage.setItem('textSizePreference', sizePreference);
}
Keyboard Navigation
If a user can’t tab through your app—they can’t use your app. Period. Ensure your HealthTech app is fully navigable without a mouse:
// Add keyboard support for custom components
document.querySelectorAll('.med-toggle-btn').forEach(button => {
button.addEventListener('keydown', (event) => {
if (event.key === ' ' || event.key === 'Enter') {
event.preventDefault();
toggleMedicationStatus(button.dataset.medicationId);
const isPressed = button.getAttribute('aria-pressed') === 'true';
button.setAttribute('aria-pressed', !isPressed);
}
});
});
Implementation Strategies
This is especially important when working with custom components.
WCAG Compliance Implementation
The WCAG framework (Web Content Accessibility Guidelines) helps ensure apps are usable by people with diverse abilities. Here’s how to implement the four core principles:
Perceivable
Provide text alternatives for visual content. Generate descriptive alt text for medication images that include important visual characteristics like color, shape, and dosage information.
Operable
Allow users to adjust timing for critical interactions. Implement customizable timers for important actions with clear visual countdowns and options to extend time limits, particularly beneficial for users who need more time to process information.
Understandable
Create clear error identification systems. When form validation fails, provide specific error messages, visually highlight problematic fields, and use proper ARIA attributes to ensure screen readers announce the errors correctly.
Robust
Develop content that works across different browsers and assistive technologies. Use well-structured, semantically correct HTML that clearly defines relationships between elements and maintains proper hierarchy.
Testing Accessibility
Even with the best intentions, accessibility issues can slip through—unless you test like it matters, something we learned while developing the Villa Medica AI-powered healthcare transformation.
Automated Testing
import { axe } from 'jest-axe';
describe('Medication Form', () => {
it('should not have accessibility violations', async () => {
document.body.innerHTML = `<form id="medication-form">...</form>`;
const results = await axe(document.body);
expect(results).toHaveNoViolations();
});
});
Screen Reader & Keyboard Testing
You can’t automate everything. Manual testing is essential—especially for:
- Screen reader flows (VoiceOver, NVDA, TalkBack)
- Keyboard navigation through all interactive elements
Responsive Design Testing
Try it zoomed. Try it rotated. Try it on an older device. That’s what your users are doing.
Implementation Strategies
Accessibility shouldn’t be a last-minute QA pass. It should be built into how your UI components work from day one.
Component Accessibility Library
Create reusable, pre-tested accessible components:
class AccessibleComponents {
static createToggleButton({id, labelText, initialState, onChange}) {
const button = document.createElement('button');
button.id = id;
button.setAttribute('aria-pressed', initialState ? 'true' : 'false');
button.textContent = labelText;
button.addEventListener('click', () => {
const newState = button.getAttribute('aria-pressed') !== 'true';
button.setAttribute('aria-pressed', newState ? 'true' : 'false');
if (onChange) onChange(newState);
});
return button;
}
}
Focus Management Utility
Create a utility for managing focus in single-page applications:
const FocusManager = {
previousFocus: null,
saveFocus() {
this.previousFocus = document.activeElement;
},
restoreFocus() {
if (this.previousFocus?.focus) {
this.previousFocus.focus();
this.previousFocus = null;
}
},
trapFocus(containerId) {
const container = document.getElementById(containerId);
if (!container) return;
const focusableElements = container.querySelectorAll(
'button, [href], input, select, textarea, [tabindex]:not([tabindex="-1"])'
);
if (focusableElements.length === 0) return;
const firstElement = focusableElements[0];
const lastElement = focusableElements[focusableElements.length - 1];
firstElement.focus();
// Handle tab key navigation
container.addEventListener('keydown', (e) => {
if (e.key !== 'Tab') return;
if (e.shiftKey && document.activeElement === firstElement) {
e.preventDefault();
lastElement.focus();
} else if (!e.shiftKey && document.activeElement === lastElement) {
e.preventDefault();
firstElement.focus();
}
});
}
};
Adaptive Interface Design
Develop interfaces that adapt to user preferences:
class AdaptiveInterface {
constructor() {
this.preferences = {
fontSize: localStorage.getItem('fontSize') || 'medium',
colorScheme: localStorage.getItem('colorScheme') || 'default',
reducedMotion: localStorage.getItem('reducedMotion') === 'true' || false,
highContrast: localStorage.getItem('highContrast') === 'true' || false
};
this.applyPreferences();
// Watch for system preference changes
this.setupMediaQueryListeners();
}
}
Technical Recap: What to Build and Why
Imagine your product is live. A caregiver logs into the app on their tablet to update a loved one’s medication schedule—with one hand, while holding a cup of tea in the other. The buttons are large enough to tap confidently. They don’t need to squint—the font size adjusts to match their system preferences. The error messages are clear. The actions are labeled. They can do what they came to do.
Now picture someone else. A visually impaired patient opens the same app using a screen reader. Every button has a proper label. The navigation is logical. The app doesn’t trap them in modals or drop focus unpredictably. The text alternatives make sense. They get through the task—independently.
This is what accessible software looks like in production. And under the hood, here’s how it’s built:
- Semantic HTML creates a structure that’s understandable and screen-reader friendly
- ARIA roles are used only where native elements don’t suffice—no more, no less
- Keyboard support is built in, with every interaction operable using
Tab
,Enter
, andSpace
- Tap targets are comfortably large, spaced to avoid misclicks—even on small screens or with shaky hands
- Layouts flex with user preferences—
rem
,%
, andmin-content
are doing the work, not pixel locks - Components are modular, tested, and accessible by design—not hacked together after QA flags something
- Automated a11y tests run on CI. Manual screen reader flows are part of routine testing.
It’s not glamorous. But it’s dependable, inclusive, and production-ready.
Accessibility is infrastructure. Treat it like versioning, testing, or auth. It’s foundational.
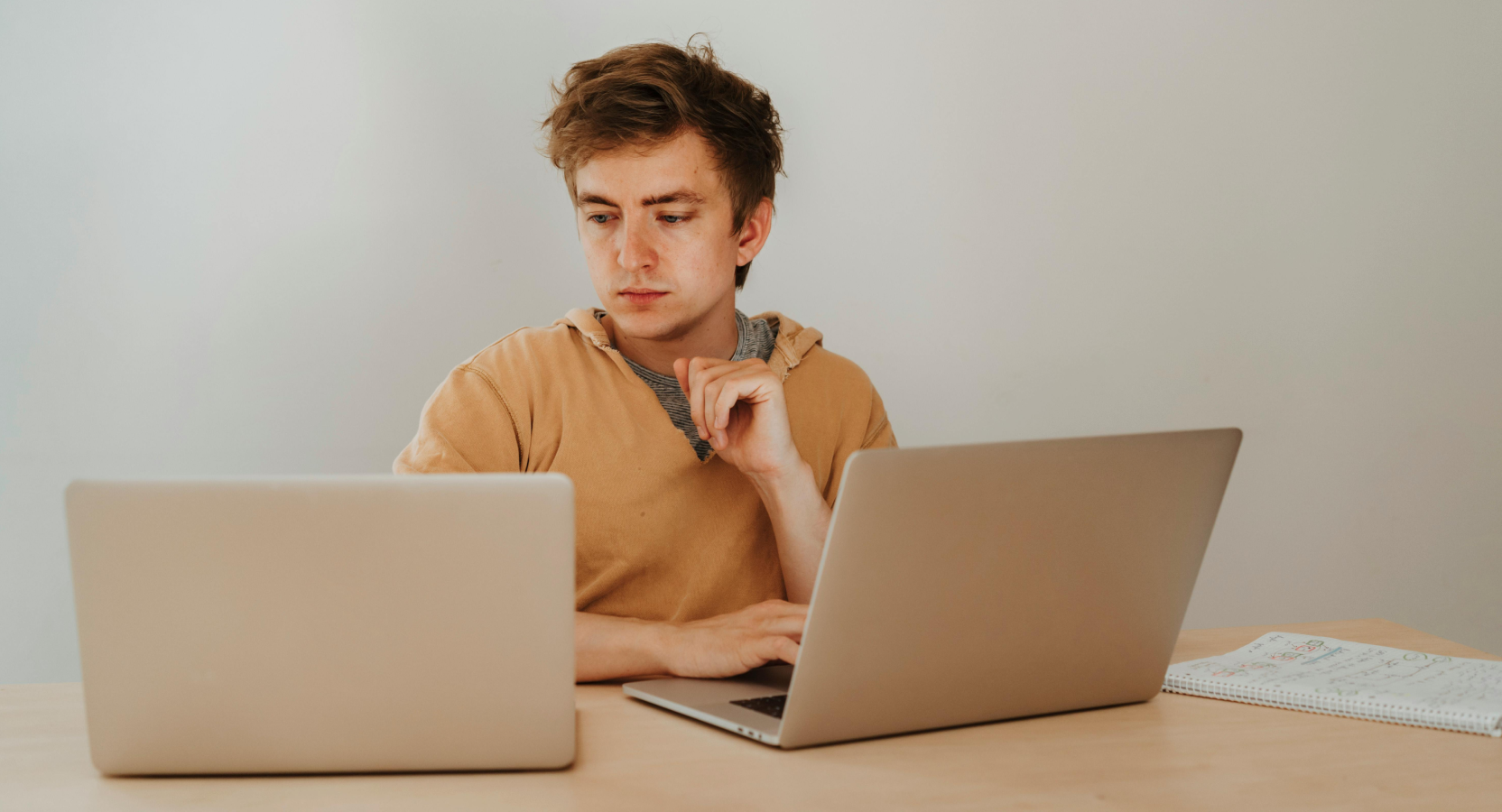
A man sits at a table with two laptops, focused on his work in a healthcare setting.
Final Thoughts
Accessibility in HealthTech isn’t a side feature. It’s a build requirement. These aren’t theoretical guidelines—they’re proven implementation details used in real-world apps that serve aging populations and users with disabilities.
Building accessible healthcare software isn't just about compliance—it's about creating products that work for everyone, including caregivers, aging users, and people with disabilities. These code-level implementation details can help transform your development approach from theoretical accessibility to practical inclusivity.
Whether you're starting a new project or looking to enhance an existing application, our team of developers has experience implementing these accessibility patterns in real-world HealthTech projects. Let's discuss how we can help make your healthcare solution more accessible and user-friendly for all.
Frequently Asked Questions
Accessibility ensures that patients, caregivers, and clinicians of all abilities can use your app. In HealthTech, this isn’t just a UX best practice—it’s a patient safety and legal requirement, especially when apps are part of clinical workflows or regulated as medical devices.
Most HealthTech apps should meet WCAG 2.1 Level AA standards. In the U.S., this also aligns with Section 508 and ADA requirements. In the EU, it’s tied to EN 301 549. These standards cover keyboard navigation, screen reader support, contrast ratios, and more.
Screen readers convert on-screen text into speech or braille. For them to work well, developers must use semantic HTML, provide proper ARIA labels, and avoid relying solely on visual cues. In clinical apps, accessible screen flows can directly impact usability and safety.
Common issues include poor contrast, missing alt text, unlabeled form fields, and custom components that don’t support keyboard navigation. These flaws can make critical health tools unusable for people with disabilities.
Yes—or they risk costly rework, failed pilots, or even non-compliance with legal standards. Accessibility should be considered from the wireframe stage, not retrofitted later. Especially in healthcare, inclusive design is a must-have, not a nice-to-have.
In most cases, yes. Public-facing apps and regulated digital health products must comply with accessibility laws in their target regions. Lack of compliance can lead to lawsuits, blocked procurement, or app store rejections.
Accessibility is built into our development process—not added as an afterthought. We follow WCAG guidelines, conduct manual and automated audits, and test with real assistive technologies to ensure inclusive, compliant HealthTech products from MVP to scale.

Let's Create the Future of Health Together
Looking for a partner who not only understands your challenges but anticipates your future needs? Get in touch, and let’s build something extraordinary in the world of digital health.